- How To Create A File Folder
- How To Make Files Into Zip Folder
- How To Create A Zip Drive Folder
- Add Folders To Zip File
The ZIP archives are used to compress and keep one or more files or folders into a single container. A ZIP archive encapsulates the files and folders as well as holds their metadata information. The most common usage of archiving is reducing the size of the files for storage or transmission and applying encryption for security. Apart from the file compression tools, the automated compression/extraction features are also used within various desktop and web applications for uploading, downloading, sharing, or encrypting the files. This article also targets similar scenarios and presents some easy ways of compressing files or folders and creating ZIP archivesprogrammatically using C#.
In this article, you will learn how to perform the following ZIP archiving operations:
If you want to zip just one file or folder, skip to step 2. Otherwise, there are two ways to select multiple files and folders: To select a consecutive group of files or folders, click the first. Zipped (compressed) files take up less storage space and can be transferred to other computers more quickly than uncompressed files. In Windows, you work with zipped files and folders in the same way that you work with uncompressed files and folders. Combine several files into a single zipped folder to more easily share a group of files. Windows: Create a folder. The quickest way to create a zip file is to place all of the files that you.
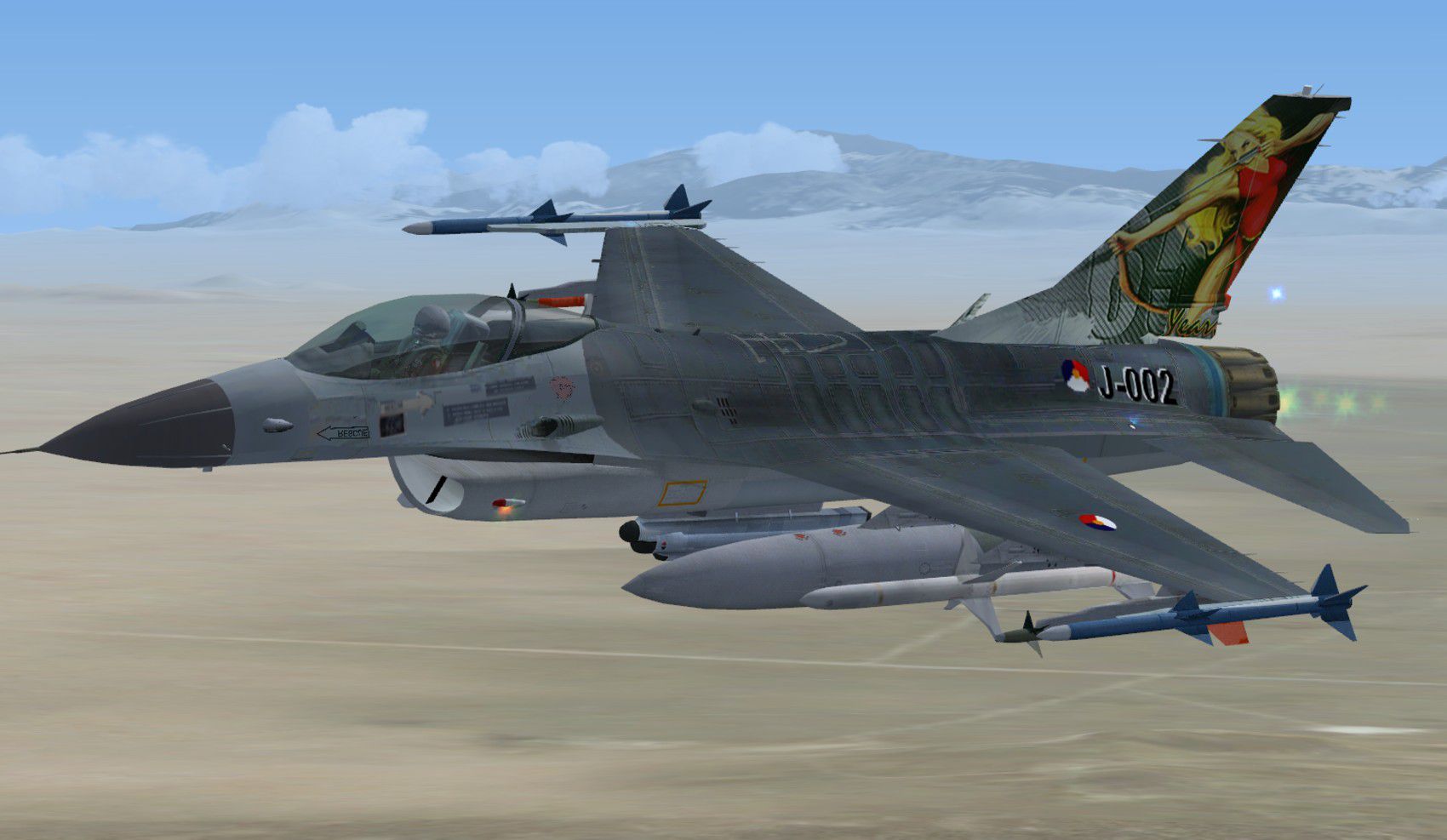
- Create a ZIP archive using C#.
- Add multiple files to a ZIP archive.
- Add folders to a ZIP archive.
- Create a password-protected ZIP archive.
- Encrypt ZIP archive with AES encryption.
Prerequisite – C# ZIP Library
Aspose.ZIP for .NET is a powerful and easy to use API for zipping or unzipping files and folders within .NET applications. It also provides AES encryption techniques to encrypt the files in ZIP archives. You can install the API from NuGet or download its binaries from the Downloads section.
Create a ZIP Archive in C#
The following are the steps to compress a file by adding it into a ZIP archive:
- Create a FileStream object for the output ZIP archive.
- Open the source file into a FileStream object.
- Create an object of Archive class.
- Add the file into the archive using Archive.CreateEntry(string, FileStream) method.
- Create the ZIP archive using Archive.Save(FileStream) method.
The following code sample shows how to add a file into a ZIP archive using C#.
Add Multiple Files into ZIP Archive in C#
In case you want to add multiple files into a ZIP archive, you can do it using one of the following ways.
ZIP Multiple Files using FileStream
In this method, the FileStream class is used to add files to the ZIP archive using Archive.CreateEntry(String, FileStream) method. The following code sample shows how to add multiple files into a ZIP in C#.
ZIP Multiple Files using FileInfo
You can also use the FileInfo class for adding multiple files into a ZIP archive. In this method, the files will be loaded using the FileInfo class and added to the ZIP archive using Archive.CreateEntry(String, FileInfo) method. The following code sample shows how to ZIP multiple files using the FileInfo class in C#.
Add Folders to a ZIP Archive in C#
You can ZIP a folder as well which could be another alternative of adding multiple files to a ZIP archive. Just put the source files into a folder and add that folder to the ZIP archive. The following are the steps to ZIP a folder:
- Create an object of FileStream class for the output ZIP archive.
- Create an instance of the Archive class.
- Use the DirectoryInfo class to specify the folder to be zipped.
- Use Archive.CreateEntries(DirectoryInfo) method to add folder into ZIP.
- Create the ZIP archive using Archive.Save(FileStream) method.
The following code sample shows how to add a folder to ZIP in C#.
Create a Password-Protected ZIP Archive in C#
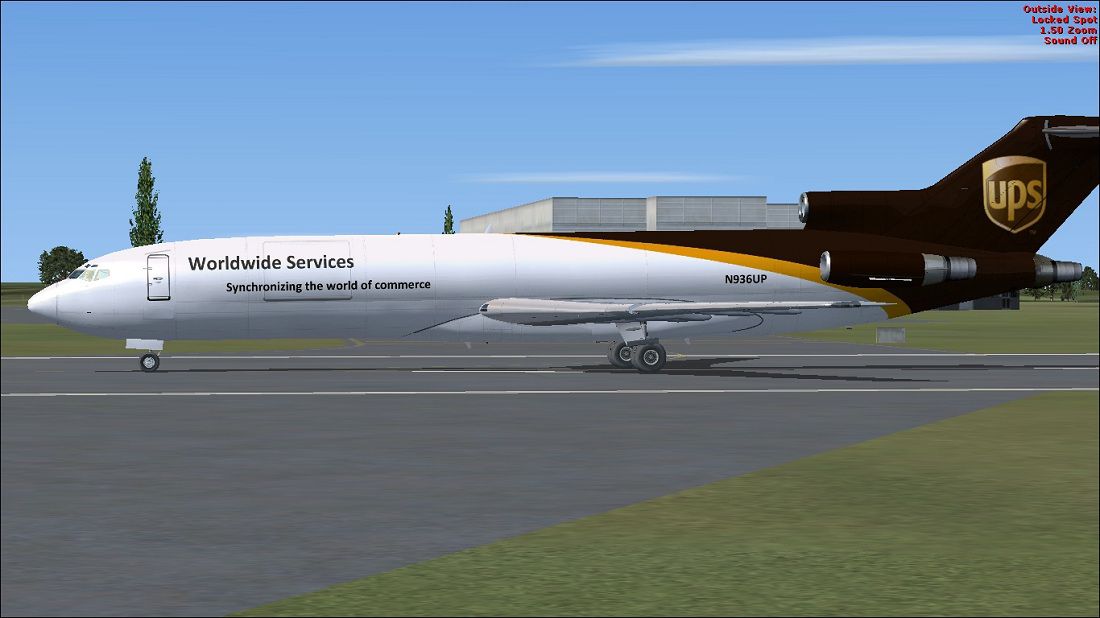
- Create a ZIP archive using C#.
- Add multiple files to a ZIP archive.
- Add folders to a ZIP archive.
- Create a password-protected ZIP archive.
- Encrypt ZIP archive with AES encryption.
Prerequisite – C# ZIP Library
Aspose.ZIP for .NET is a powerful and easy to use API for zipping or unzipping files and folders within .NET applications. It also provides AES encryption techniques to encrypt the files in ZIP archives. You can install the API from NuGet or download its binaries from the Downloads section.
Create a ZIP Archive in C#
The following are the steps to compress a file by adding it into a ZIP archive:
- Create a FileStream object for the output ZIP archive.
- Open the source file into a FileStream object.
- Create an object of Archive class.
- Add the file into the archive using Archive.CreateEntry(string, FileStream) method.
- Create the ZIP archive using Archive.Save(FileStream) method.
The following code sample shows how to add a file into a ZIP archive using C#.
Add Multiple Files into ZIP Archive in C#
In case you want to add multiple files into a ZIP archive, you can do it using one of the following ways.
ZIP Multiple Files using FileStream
In this method, the FileStream class is used to add files to the ZIP archive using Archive.CreateEntry(String, FileStream) method. The following code sample shows how to add multiple files into a ZIP in C#.
ZIP Multiple Files using FileInfo
You can also use the FileInfo class for adding multiple files into a ZIP archive. In this method, the files will be loaded using the FileInfo class and added to the ZIP archive using Archive.CreateEntry(String, FileInfo) method. The following code sample shows how to ZIP multiple files using the FileInfo class in C#.
Add Folders to a ZIP Archive in C#
You can ZIP a folder as well which could be another alternative of adding multiple files to a ZIP archive. Just put the source files into a folder and add that folder to the ZIP archive. The following are the steps to ZIP a folder:
- Create an object of FileStream class for the output ZIP archive.
- Create an instance of the Archive class.
- Use the DirectoryInfo class to specify the folder to be zipped.
- Use Archive.CreateEntries(DirectoryInfo) method to add folder into ZIP.
- Create the ZIP archive using Archive.Save(FileStream) method.
The following code sample shows how to add a folder to ZIP in C#.
Create a Password-Protected ZIP Archive in C#
You can protect the ZIP archives using the password. In order to specify a password for the ZIP archive, the ArchiveEntrySettings class is used in the constructor of the Archive. The following code sample shows how to create a password-protected ZIP archive in C#.
Create AES Encrypted ZIP Archives in C#
Aspose.ZIP for .NET also lets you apply AES encryption to the ZIP archives. You can use the following AES encryption methods:
- AES128
- AES192
- AES256
In order to apply AES encryption, API offers the AesEcryptionSettings class. The following code sample shows how to create an AES encrypted ZIP archive in C#. How take a screenshot on computer.
Learn more about Aspose.ZIP for .NET
Explore more about our C# ZIP API using the following resources:
See also
With PDF Converter app, you can quickly convert ZIP to PDF files without even extracting the contents from it. Here is how it is done.
Simply right-click the ZIP file in your Windows Explorer, and click ‘Instant .pdf' menu.
By default, the app will automatically extract the contents of the ZIP and convert each of the file to PDF. Consequently, it places the converted PDF files in the same folder as that of the ZIP file. However, you can change this preference, to output the converted files to a subfolder, having the same name as that of the ZIP file.
Furthermore, if you want more control over the PDF output, such as changing the sequence or order of the files, or exclude certain files of the ZIP, click ‘Convert to PDF (Advanced)…‘ menu.
How To Create A File Folder
In the advanced mode, firstly, you can choose the PDF output mode for all files inside the Zip file. For instance, if to convert each file to own PDF file, or combine all files to one PDF file. Secondly, you can specify the PDF paper type, orientation or destination output folder, or apply watermark. Finally, you can set passwords, security permissions such as preventing the PDF from opening, printing, copying or editing etc.
Combine files under Zip to One PDF
Multiple documents in a ZIP file may be related and about a project, client or entity. Such documents naturally belong together and combining all of them makes it easy to see their relationship. And PDF Converter makes it simple and quick to combine such documents into one PDF file, in a click. All you need to do is right-click the ZIP file, and press ‘Combine to One Pdf‘ from the context menu. As a result, this will combine all the files and documents inside the ZIP into one PDF file.
How To Make Files Into Zip Folder
And what you get is a PDF file, containing all the inner files/folders or even zip files – all merged into one long, continuous PDF file. In addition, it adds table of contents or bookmarks to the PDF file. As can be seen below, this lets you to quickly jump to the individual document within the PDF file.
Summary
The converter supports more than 120 files types including a ZIP file, for seamless conversion to PDF without external dependencies. All in all, PDF Converter app is the go-to solution for all your PDF conversion requirements for Windows platform.
How To Create A Zip Drive Folder
NOTE: A compressed ‘ZIP' file packs one or more files or folders into a single file and takes up less space. A compressed zip file can be a very useful way of sending or storing files. You'll need to unzip them to extract the contents inside and work with the inner files.
Add Folders To Zip File
** Microsoft and the Office logo are trademarks or registered trademarks of Microsoft Corporation in the United States and/or other countries.